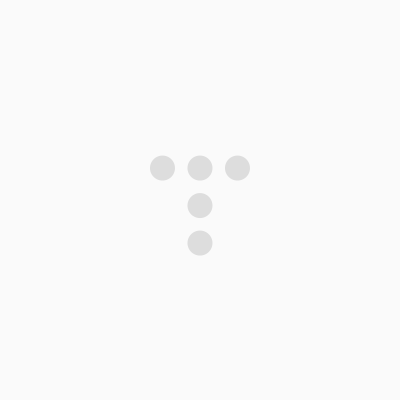
[Day-8] 상품 엔티티, 리파지토리, 서비스WEB JAVA SPRING/PROJECT2023. 8. 25. 16:10
Table of Contents
<상품 도메인 개발>
구현 기능
- 상품 등록
- 상품 목록 조회
- 상품 수정
#파일구조
#상품 엔티티 개발(비즈니스 로직 추가)
domain -> Item
//비즈니스 로직
/*
재고 증가
*/
public void addStock(int quantity){
this.stockQuantity+=quantity;
}
/*
재고 감소
*/
public void removeStock(int quantity){
int restStock = this.stockQuantity -quantity;
if(restStock <0 ){
throw new NotEnoughStockException("need more stock");
}
this.stockQuantity =restStock;
}
NotEnoughStockException
public class NotEnoughStockException extends RuntimeException {
public NotEnoughStockException() {
super();
}
public NotEnoughStockException(String message) {
super(message);
}
public NotEnoughStockException(String message, Throwable cause) {
super(message, cause);
}
public NotEnoughStockException(Throwable cause) {
super(cause);
}
}
#상품 리파지토리 개발
itemRepository
@Repository
@RequiredArgsConstructor
public class ItemRepository {
private final EntityManager em;
//item은 jpa에 저장하기 전까지 id값이 없다(=완전히 새로 생성하는 객체)
public void save(Item item) {
if ((item.getId() == null)) {
em.persist(item); //상품 신규 등록
} else { //만약에 db에 이미 있는 값이라면 수정
em.merge(item);
}
}
public Item findOne(Long id) {
return em.find(Item.class, id);
}
public List<Item> findAll() {
return em.createQuery("select i from Item i",Item.class).getResultList();
}
}
#상품 서비스 개발
itemService
상품 리포지토리에 단순히 위임만 하는 클래스
@Service
@Transactional(readOnly = true)
@RequiredArgsConstructor
public class itemService {
private final ItemRepository itemRepository;
@Transactional
public void saveItem(Item item) {
itemRepository.save(item);
}
public List<Item> findItems() {
return itemRepository.findAll();
}
public Item findOne(Long itemId) {
return itemRepository.findOne(itemId);
}
}
'WEB JAVA SPRING > PROJECT' 카테고리의 다른 글
[Day-10] 주문기능테스트, 부트스트랩적용 (0) | 2023.08.25 |
---|---|
[Day-9] 주문 엔티티, 리파지토리, 서비스 (0) | 2023.08.25 |
[Day-7] 회원 도메인, 리포지토리, 서비스, 회원 기능 테스트 (0) | 2023.08.25 |
[Day-6] 엔티티 설계 (연관관계) (0) | 2023.08.25 |
[Day-5] 엔티티 클래스 개발2 (0) | 2023.08.25 |