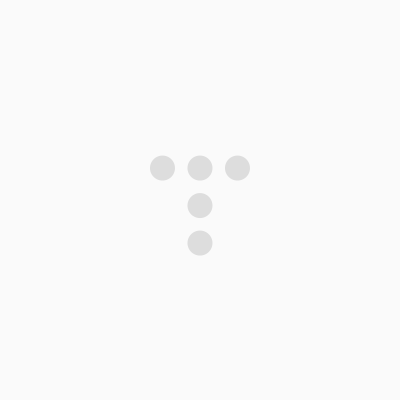
jqueryWEB JAVA SPRING/JS2023. 9. 12. 17:22
Table of Contents
제이쿼리와 Ajax
Ajax를 이용하여 개발을 손쉽게 할 수 있도록 미리 여러 가지 기능을 포함해 놓은 개발 환경을
Ajax 프레임워크라고 합니다.
그중에서도 현재 가장 널리 사용되고 있는 Ajax 프레임워크는 바로 제이쿼리(jQuery)입니다.
$.ajax({
url: "/examples/media/request_ajax.php", // 클라이언트가 요청을 보낼 서버의 URL 주소
data: { name: "홍길동" }, // HTTP 요청과 함께 서버로 보낼 데이터
type: "GET", // HTTP 요청 방식(GET, POST)
dataType: "json" // 서버에서 보내줄 데이터의 타입
})
// HTTP 요청이 성공하면 요청한 데이터가 done() 메소드로 전달됨.
.done(function(json) {
$("<h1>").text(json.title).appendTo("body");
$("<div class=\"content\">").html(json.html).appendTo("body");
})
// HTTP 요청이 실패하면 오류와 상태에 관한 정보가 fail() 메소드로 전달됨.
.fail(function(xhr, status, errorThrown) {
$("#text").html("오류가 발생했습니다.<br>")
.append("오류명: " + errorThrown + "<br>")
.append("상태: " + status);
})
// HTTP 요청이 성공하거나 실패하는 것에 상관없이 언제나 always() 메소드가 실행됨.
.always(function(xhr, status) {
$("#text").html("요청이 완료되었습니다!");
});
간단한 예제
$(function() {
$("#requestBtn").on("click", function() {
$.ajax("/examples/media/request_ajax.php")
.done(function() {
alert("요청 성공");
})
.fail(function() {
alert("요청 실패");
})
.always(function() {
alert("요청 완료");
});
});
});
http://www.tcpschool.com/ajax/ajax_jquery_ajax
실습코드
exam01
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<!-- <script src="js/jquery-3.6.0.min.js"></script> -->
<!-- <script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script> -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
window.onload = function() {
alert('load...')
}
//위에거 쓰기 싫어서
$(document).ready(function() {
alert('ready...')
})
$(document).ready(function() {
alert('ready2...')
})
$(function() { //축약형
alert('ready3...')
})
</script>
</head>
<body>
</body>
</html>
exam02
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function(){
alert('ready')
//#id .class
//$('h1').hide() //요소 숨김
//$('#h01').hide() //h1의 id h01인것만 숨김
//$('.c01').hide()
//$('h1.c01').hide()
//$('body > * ').hide() //body 밑에 있는 모든 후손들
//$('h1:first').hide() // h1의 첫번째거 숨겨
$('h1:last').hide()
/*
자바스크립트로 쓰면 불편
let h1Tags= document.getElementsByTagName("h1")
for(let tag of h1Tags){
tag.style.display='none'
}
*/
})
</script>
</head>
<body>
<hr>
<h1 id="h01">Hello</h1>
<h1 class="c01">Hi</h1>
<h2>good-bye</h2>
<hr>
<h1>Hello</h1>
<h1 class="c01">Hi</h1>
<h2 class="c01">good-bye</h2>
<hr>
</body>
</html>
exam03
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function(){
alert('dd')
//$('ul li').hide() //ul밑에 li 숨김
//$('ul li:first').hide() //ul밑에 li의 첫번째것 숨김 ->호랑이
//$('ul li:last').hide() // 마지막 짜장면 지워짐
//$('ul li:first-child').hide() //호랑이 떡볶이 지워짐
$('ul li:last-child').hide() //코끼리 짜장면
})
</script>
</head>
<body>
<h1>동물종류</h1>
<ul>
<li>호랑이</li>
<li>사자</li>
<li>코끼리</li>
</ul>
<h1>음식종류</h1>
<ul>
<li>떡볶이</li>
<li>라면</li>
<li>순대</li>
<li>짜장면</li>
</ul>
</body>
</html>
exam04
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js">
</script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js" integrity="sha256-T0Vest3yCU7pafRw9r+settMBX6JkKN06dqBnpQ8d30=" crossorigin="anonymous"></script>
<script>
$(document).ready(function() {
//alert('d')
//$('a').hide()
//$('a[target]').hide() //다음 사라짐
//$('a[href="http://www.naver.com"]').hide() //네이버 사라짐
//$('input[type=text]').hide()
//$('input:text').hide() //이런건 input태그만 가능함 text인거 숨김
//$('input:button').hide() //버튼 1
//$('button').hide() // 버튼2
//$(':text').hide() // type이 text 숨겨라
//$(':button').hide() //
//버튼클릭->함수호출
$(':button').click(function(){
//$('hr').hide() //선사라짐
$(this).hide("drop" , { direction: "down" }, "slow") //버튼 사라짐
})
})
</script>
</head>
<body>
<h2>a Tag전</h2>
<a href="http://www.naver.com">네이버</a>
<a href="http://www.daum.net" target="_blank">다음</a>
<h2>a Tag후</h2>
<hr>
<input type="text">
<input type="button" value="버튼 1">
<button>버튼2</button>
<hr>
</body>
</html>
exam05
<script>
$(document).ready(function() {
$('#menu').hover(function() {
//$('#submenu').slideDown(1000)
$('#submenu').slideToggle(1000)
}, function() {
//$('#submenu').slideUp(1000)
$('#submenu').slideToggle(1000)
})
})
</script>
</head>
<body>
<div id='menu'>마우스 접근시켜보세요</div>
<div id='submenu'>메뉴화면</div>
</body>
</html>
exam06
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style>
h1 {
display: none
}
</style>
<script src="js/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
/*
3초에 걸려서 Hello World 문장이 화면에 보인 뒤, complete 메세지를 경고창에 띄우기
*/
// $('h1').show(3000)
// alert('complete...')
/* $('h1').show(3000, function() {
alert('complete...')
}) */
//3초에 걸려서 Hello World 문장이 화면에 보인 뒤, 글자색 파란색 -> 슬라이드 효과 ->배경색 노란색
$('h1').show(3000, function() {
$(this).css('color','blue')
$(this).slideUp('slow').slideDown('slow',function(){
$(this).css('background-color','yellow')
})
})
})
/*
[ $(this) - Jquery]
Jquery의 경우에는 이벤트가 발생한 요소의 정보들이 Object로 표시
this ==$(this)[0]
*/
</script>
</head>
<body>
<h1>Hello World</h1>
</body>
</html>
exam07
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js"> </script>
<script>
$(document).ready(function(){
//h1태그에 있는거 클릭할때마다 이벤트 발생
$('h1').click(function(event,){
$(this)[0].innerText+='*' //문자열에 계속추가
})
setInterval(function(){
//$('h1').click()
$('h1').trigger('click')
},1000)
})
//수행률 : *********************
</script>
</head>
<body>
<h1> 수행률 : </h1>
</body>
</html>
exam08
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js"> </script>
<script>
$(document).ready(function(){
$('button').click(function() {
//console.log($('#spanObj')[0])
let spanTag=$('#spanObj')[0] //span id가 spanObj인거의 첫번째요소
spanTag.innerHTML = parseInt(spanTag.innerHTML) + 1
/* let spanTag = document.getElementById("spanObj")
spanTag.innerHTML = parseInt(spanTag.innerHTML) + 1
*/
})
})
</script>
</head>
<body>
<span id="spanObj">0</span>번 클릭
<button>클릭</button>
</body>
</html>
exam09
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
/* let pTags = document.getElementsByTagName("p");
let html =pTags[0].innerHTML
let text =pTags[0].innerText
*/
let html = $('p#p01').html() // 이자식 냅다 태그 까지 출력하는 멍청이
let text = $('p').text() //텍스트만 나옴
alert('추출된 html :' + html + '\n추출된 text :' + text)
let aTag = document.getElementsByTagName("a")[0]
//let href = aTag.href
//let href = aTag.getAttribute('href')
let href = $('a').attr('href') //attr(): 속성값 가져옴 =>a 가져옴
alert('추출된 href :' + href)
//버튼누를때마다 클릭 함수 고
$('button').click(function() {
/* let inputTag = document.getElementByID("name")
let name = inputTag.value
*/
let name = $('#name').val() //아이디name인거 선택한 양식의 값을 가져와==val
alert('입력된 이름 : ' + name)
})
})
</script>
</head>
<body>
<p id="p01">내이름은 <b>홍길동</b>이고 , 별명은 <i>의적 </i>입니다</p>
<p>내이름은 <b>홍길순</b>이고 , 별명은 <em>의적 </em>입니다</p>
<a href="http://www.naver.com">네이버</a><br>
이름 : <input type="text" name="name" id="name"> //아이디 여기
<button>입력완료</button>
</body>
</html>
exam10
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
//btn01이 아이디인거, 클릭하면
$('#btn01').click(function() {
$('#p01').text('<mark>문장이 변환되었습니다</mark>')
})
$('#btn02').click(function() {
$('#p02').html('<mark>문장이 변환되었습니다</mark>')
})
$('#btn03').click(function() {
$('a').attr('href', 'http://www.daum.net') //a태그 속성바꿈
})
$('#btn04').click(function() {
// $('p').html('문장변환')
$('p').html(function(index, element) {
//console.log(index, element)
return index + ' : <strong>문장변환</strong>'
})
})
})
</script>
</head>
<body>
<a href="http://www.naver.com" target="_blank">사이트이동</a>
<p id="p01">첫번째 문장입니다</p>
<p id="p02">두번째 문장입니다</p>
<button id="btn01">TEXT변환</button>
<button id="btn02">HTML변환</button>
<button id="btn03">주소변환</button>
<button id="btn04">문장변환</button>
</body>
</html>
exam11
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="js/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$('#btn01').click(function() {
/*
// <h1>세번째문장</h1>
let h1Tag = document.createElement('h1')
let text = document.createTextNode('세번째 문장')
h1Tag.appendChild(text)
// console.log(h1Tag)
document.querySelector('div').appendChild(h1Tag)
*/
$('div').append('<h1>세번째 문장</h1>')
})
$('#btn02').click(function() {
/* $('ol').append('<li>노랑</li>') */
$('ol').prepend('<li>노랑</li>')
})
})
$(document).ready(function() {
$('#btn03').click(function() {
// $('body').prepend('<h4>추가된 문장</h4>')
// $('body').prepend('<h3>또 추가된 문장</h3>')
// $('body').prepend('<h6>또또 추가된 문장</h6>')
$('body').prepend('<h4>추가된 문장</h4>',
'<h3>또 추가된 문장</h3>','<h6>또또 추가된 문장</h6>')
})
})
</script>
</head>
<body>
<div>
<h1>첫번째 문장</h1>
<h1>두번째 문장</h1>
<!-- <h1>세번째 문장</h1> -->
</div>
<h2>색상표</h2>
<ol>
<li>빨강</li>
<li>파랑</li>
<li>초록</li>
<!--ol은 순서있어서 1.2.3. 으로 찍힘 -->
</ol>
<button id="btn01">문장추가</button>
<button id="btn02">색상추가</button>
<button id="btn03">본문추가</button>
</body>
</html>
<!--
<<<ul,ol태그>>>
ul : unorder list -> 순서가 없는 리스트
ol :order list -> 순서 있음
그래서 안에 li 무조건 있어야함 ->list item
-->
exam12
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style>
img {
width: 200px;
height: 200px;
float: left;
}
button {
height: 200px;
float: left;
}
</style>
<script src="http://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$('#next').click(function() {
$('img').last().prependTo('#gallery')
})
//-> 이동
/* $('#prev').click(function() {
$('img').first().appendTo('#gallery')
})
//< */
//주기적으로 인자실행
setInterval(function() {
//이벤트가 걸리지 않았지만 강제로 걸음->계속클릭
$('#next').trigger('click')
}, 2000)
})
</script>
</head>
<body>
<button id="prev">PREV</button>
<span id="gallery">
<img src="/Lecture-Web/html/images/dog2.jpg">
<img src="/Lecture-Web/html/images/dog3.jpg">
<img src="/Lecture-Web/html/images/cat.gif">
<img src="/Lecture-Web/html/images/pencil.png">
</span>
<button id="next">NEXT</button>
</body>
</html>
'WEB JAVA SPRING > JS' 카테고리의 다른 글
JSON, JavaScript 객체 차이 (0) | 2023.10.05 |
---|---|
Ajax 개념과 코드 적용(댓글 등록) (0) | 2023.09.12 |
json데이터를 활용한 기초적인 홈페이지 (0) | 2023.08.24 |
자바스크립트 테이블 만들기 (0) | 2023.08.24 |
자바스크립트 과목,평균,반평균 점수,순위 출력하기 (0) | 2023.08.24 |